Model-View-Controller (MVC) is an architectural pattern we all are well aware of. It’s a de-facto standard for almost all UI and Web frameworks. It is convenient and easy to use. It is simple and effective. It is a great concept … for a procedural programmer. If your software is object-oriented, you should dislike MVC as much as I do. Here is why.
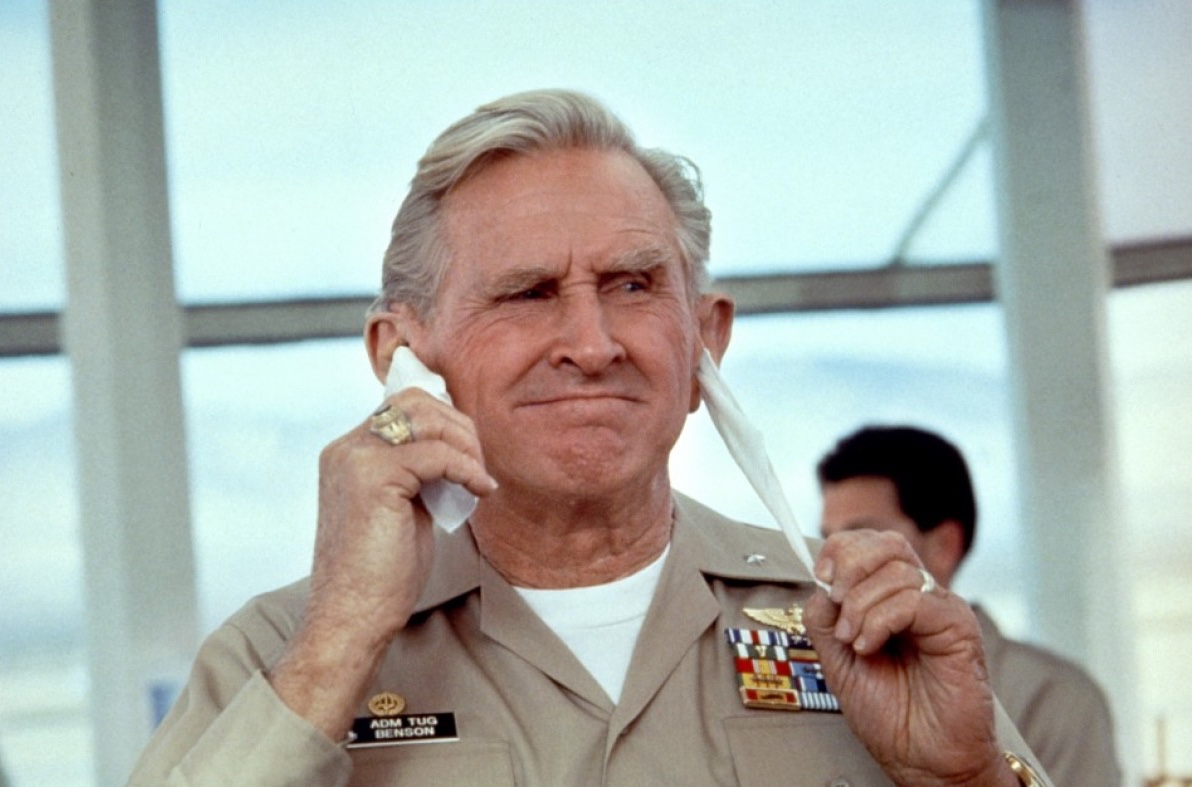
This is how MVC architecture looks:
Controller is in charge, taking care of the data received from Model and injecting it into View—and this is exactly the problem. The data escapes the Model and becomes “naked,” which is a big problem, as we agreed earlier. OOP is all about encapsulation—data hiding.
MVC architecture does exactly the opposite by exposing the data and hiding behavior. The controller deals with the data directly, making decisions about its purpose and properties, while the objects, which are supposed to know everything about the data and hide it, remain anemic. That is exactly the principle any procedural architecture is built upon; the code is in charge of the data. Take this C++ code, for example:
void print_speed() { // controller
int s = load_from_engine(); // model
printf("The speed is %d mph", s); // view
}
The function print_speed()
is the controller. It gets the data s
from the model load_from_engine()
and renders it via the view printf()
. Only the controller knows that the data is in miles per hour. The engine returns int
without any properties. The controller simply assumed that that data is in mph. If we want to create a similar controller somewhere else, we will have to make a similar assumption again and again. That’s what the “naked data” problem is about, and it leads to serious maintainability issues.
This is an object-oriented alternative to the code above (pseudo-C++):
printf(
new PrintedSpeed( // view
new FormattedSpeed( // controller
new SpeedFromEngine() // model
)
)
);
Here, SpeedFromEngine.speed()
returns speed in mph, as an integer; FormattedSpeed.speed()
returns "%d mph"
; and finally, PrintedSpeed.to_str()
returns the full text of the message. We can call them “model, view, and controller,” but in reality they are just objects decorating each other. It’s still the same entity—the speed. But it gets more complex and intelligent by being decorated.
We don’t tear the concept of speed apart. The speed is the speed, no matter who works with it and where it is presented. It just gets new behavior from decorators. It grows, but never falls apart.
To summarize, Controller is a pure procedural component in the MVC trio, which turns Model into a passive data holder and View into a passive data renderer. The controller, the holder, the renderer … Is it really OOP?